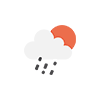
Please enable JavaScript to use this page!
This page is designed to help alleviate the stress and uncertainty of those very questions. At its core computer code is simply 'instructions' we programmers type for the computers to do certain 'tasks'. They can be tasks with numbers or tasks with words or pictures or interacting with other things on the internet or any combination of these things. While Java, Python, and JavaScript are very different they also share many things in common. Before we dive into the code let's explore these languages. At first glance it would seem that the 3 biggest languages currently used are as different as German, Mandarin, and Sanskrit. While this may be true in some regards, upon a deeper understanding it is more accurate to compare them to French, Spanish and Italian. While they have different ways of doing the same things and slightly different requirements to get there they have a lot in common.
These languages are all very different but have similar ways of changing numbers and words.
// bill tip
function setBill() {
let bill = document.getElementById("billAmount").value;
let tip = document.getElementById("tipPercent").value;
let amount = 0;
amount = bill * (tip / 100);
console.log(amount);
clean = "Tip amount : $ " + (Math.round(amount*100)/100).toFixed(2);
let answer = document.getElementById("tipAmount");
answer.textContent = clean;
}
// to stop page re-load while clicking 'Try me tip'
document.getElementById("billset").addEventListener("click", function (event) {
event.preventDefault();
});
import sys
while True:
print("welcome to tip clac enter the bill amount or q to exit")
bill = input()
if (bill == "q"):
sys.exit()
print("enter the percent to tip or q to exit")
percent = input()
if (percent == "q"):
sys.exit()
total = (float(percent)/100)* float(bill)
print(" for a bill of $" +bill +" tipping "+ percent +"% = $")
print(round(total, 2))
print("run again? (y)es or (q) q for quit")
again = input()
if (again == 'q'):
sys.exit()
import java.math.RoundingMode;
import java.text.DecimalFormat;
import java.util.*;
public class Main {
public static void main(String[] args) {
// write your code here
double bill = 0.00;
double tip = 0.00;
double amount = 0.00;
Scanner input = new Scanner(System.in);
boolean running = true;
while (running) {
print("Welcome to tip calc ... please input the bill amount");
bill = Double.parseDouble(input.nextLine().trim());
print("enter the percent you want to tip as a whole number");
tip = Double.parseDouble(input.nextLine().trim());
amount = bill * (tip / 100);
DecimalFormat df = new DecimalFormat("#.##");
df.setRoundingMode(RoundingMode.CEILING);
print(" for the bill $" + bill + " & tipping " + tip + " % = $" + df.format(amount) + " for the tip.");
print(" use again ? (y)es or (n)o ?");
String again = input.nextLine().toLowerCase();
if (again.startsWith("n")) {
running = false;
break;
}
}
}
public static void print(Object _O) {
System.out.println(_O);
}
}
function piglatinize() {
wordIn = document.getElementById("pigWordIn").value;
console.log(wordIn);
plWord = document.getElementById("pigWordOut");
let words = wordIn.split(" "),
newWords = [];
for (var i = 0; i < words.length; i++) {
newWords.push(this.translate(words[i]));
}
plWord.textContent = newWords.join(" ");
}
function translate(word) {
let array = word.split(""),
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"],
newWord = "";
for (var y = 0; y < word.length; y++) {
if (vowels.includes(word[y])) {
if (y === 0) {
return word + "yay";
}
return word.slice(y, word.length) + word.slice(0, y) + "ay";
}
}
}
// to stop page re-load on click pig latin
document
.getElementById("pigLatinBtn")
.addEventListener("click", function (event) {
event.preventDefault();
});
# Python program to encode a word to a Pig Latin.
def isVowel(c):
return (c == 'A' or c == 'E' or c == 'I' or
c == 'O' or c == 'U' or c == 'a' or
c == 'e' or c == 'i' or c == 'o' or
c == 'u');
def pigLatin(s):
# the index of the first vowel is stored.
length = len(s);
index = -1;
for i in range(length):
if (isVowel(s[i])):
index = i;
break;
# Pig Latin is possible only if vowels
# is present
if (index == -1):
return "-1";
# Take all characters after index (including
# index). Append all characters which are before
# index. Finally append "ay"
return s[index:] + s[0:index] + "ay";
str = pigLatin("graphic");
if (str == "-1"):
print("No vowels found. Pig Latin not possible");
else:
print(str);
# This code contributed by Rajput-Ji
// Java program to encode a word to a Pig Latin.
class GFG {
static boolean isVowel(char c) {
return (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U' ||
c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
}
static String pigLatin(String s) {
// the index of the first vowel is stored.
int len = s.length();
int index = -1;
for (int i = 0; i < len; i++)
{
if (isVowel(s.charAt(i))) {
index = i;
break;
}
}
// Pig Latin is possible only if vowels
// is present
if (index == -1)
return "-1";
// Take all characters after index (including
// index). Append all characters which are before
// index. Finally append "ay"
return s.substring(index) +
s.substring(0, index) + "ay";
}
// Driver code
public static void main(String[] args) {
String str = pigLatin("graphic");
if (str == "-1")
System.out.print("No vowels found." +
"Pig Latin not possible");
else
System.out.print(str);
}
}
// This code is contributed by Anant Agarwal.
// rps
// access the html
let playerChose = document.getElementById("player");
let playerMove = "pick";
playerChose.innerText = playerMove;
// player / you pick rock
let rockChosen = document.getElementById("rockChoice");
rockChosen.addEventListener("click", rockChoice);
function rockChoice() {
playerChose.innerText = "ROCK";
playerMove = "rock";
}
// player / you pick paper
let paperChosen = document.getElementById("paperChoice");
paperChosen.addEventListener("click", paperChoice);
function paperChoice() {
playerChose.innerText = "PAPER";
playerMove = "paper";
}
// player / you pick scissor
let scissorChosen = document.getElementById("scissorChoice");
scissorChosen.addEventListener("click", scissorChoice);
function scissorChoice() {
playerChose.innerText = "SCISSORS";
playerMove = "scissors";
}
// computers move random number 0 1 or 2
function opponetMove() {
switch (Math.floor(Math.random() * 3)) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
}
}
// the button
let play = document.getElementById("playRPS");
play.addEventListener("click", rps);
// display computers choice
let opponet = document.getElementById("opponet");
// the game
function rps(e) {
// keep window open don't re-load page
e.preventDefault();
if (playerChose.innerText === "pick") {
alert("please choose rock paper or scissors first");
} else {
// run the function when click so we get a different choice every click
let om = opponetMove();
// update html
opponet.innerText = om;
// display win lose or draw
winner(playerMove, om);
return om;
}
}
// html access
let outcome = document.getElementById("outcome");
// outcomes logic
function winner(playerMove, om) {
if (playerMove == om) {
outcome.innerText = "Tie";
} else if (playerMove == "rock" && om == "paper") {
outcome.innerText = "you lose";
} else if (playerMove == "paper" && om == "scissors") {
outcome.innerText = "you lose";
} else if (playerMove == "scissors" && om == "rock") {
outcome.innerText = "you lose";
} else {
outcome.innerText = "you WIN !";
}
}
//////////////////////////
import random
import sys
wins = 0
losses = 0
ties = 0
while True: # The main game loop.
print('%s Wins, %s Losses, %s Ties' % (wins, losses, ties))
while True: # The player input loop.
print('Enter your move: (ro)ck (pa)per (sc)issors or (q)uit')
playerMove = input()
if playerMove == 'q':
print('thanks for playing your final score was: ')
print('%s Wins, %s Losses, %s Ties' % (wins, losses, ties))
sys.exit() # Quit the program.
if playerMove in['ro','pa','sc'] :
break # Break out of the player input loop.
print('Type one of ro, pa, sc, or q.')
# Display what the player chose:
if playerMove == 'ro':
print('ROCK versus...')
elif playerMove == 'pa':
print('PAPER versus...')
elif playerMove == 'sc':
print('SCISSORS versus...')
# Display what the computer chose:
randomNumber = random.randint(1, 5)
if randomNumber == 1:
computerMove = 'ro'
print('ROCK')
elif randomNumber == 2:
computerMove = 'pa'
print('PAPER')
elif randomNumber == 3:
computerMove = 'sc'
print('SCISSORS')
# Display and record the win/loss/tie:
# start with a tie
if playerMove == computerMove:
print('It is a tie!')
ties = ties + 1
# Win
elif playerMove == 'ro' and computerMove == 'sc':
print('You win! rock crushes scissors')
wins = wins + 1
elif playerMove == 'pa' and computerMove == 'ro':
print('You win! paper covers rock')
wins = wins + 1
elif playerMove == 'sc' and computerMove == 'pa':
print('You win! scissors cuts paper')
wins = wins + 1
# lose
elif playerMove == 'ro' and computerMove == 'pa':
print('You lose! computer paper covered your rock')
losses = losses + 1
elif playerMove == 'pa' and computerMove == 'sc':
print('You lose! computer scissors cut your paper')
losses = losses + 1
elif playerMove == 'sc' and computerMove == 'ro':
print('You lose! computer rock smashed your scissors')
losses = losses + 1
import java.util.*;
public class Main {
private static void print(Object o) {
System.out.println(o);
}
public static void main(String[] args) {
// Brian's code for simple rock paper scissors
Scanner input = new Scanner(System.in); // Object for getting input from user keyboard
Random myRand = new Random(); // Object for Generating Random Numbers for comp move
// weapons
int RO = 0; // = Rock
int PA = 1; // = Paper
int SC = 2; // = Scissors
// variables
int user_choice; // User's Choice
int machine_choice; // Computer's Choice
boolean keep_playing = true;
int wins = 0;
int losses = 0;
int ties = 0;
// gameplay
while (keep_playing) {
print("Welcome to the java game of Rock Paper Scissors");
print("| ScoreBoard: your wins are = " + wins + "| your losses are = " + losses + "
| your ties are = " + ties + " |");
print("It is you vs the machine choose your weapon by its number");
print("0. Rock");
print("1. Paper");
print("2. Scissors");
user_choice = input.nextInt(); // Get user input from keyboard
machine_choice = myRand.nextInt(3); // Make a random number between 0 and 2 for the computer's choice.
// what each 'player' chose
print("Machine throws... " + machine_choice + " _-_ You picked... " + user_choice);
// results logic
if (user_choice == machine_choice) {
ties++;
print("It's a Tie Game!");
} else if ((user_choice == R && machine_choice == S) || (user_choice == S && machine_choice == P)
|| (user_choice == P && machine_choice == R)) {
wins++;
print("You the user Wins!");
} else {
losses++;
print("Sorry sport ... The Computer Wins!");
}
print("Want to continue playing ? ");
print("enter any positive number to keep going, or enter any negative number to stop");
int more = input.nextInt();
if (more < 0) {
print("ok now bye bye then ");
print("| ScoreBoard: your wins were = " + wins +
"| your losses were = " + losses + " | your ties were = " + ties + " |");
keep_playing = false;
}
}
}
}
/// click rock paper spock ect....
/// word under your move changes
/// color outline changes
/// red rock, silver scissors, white paper, blue spock, green lizard
/// press button for comps move
/// score and status updates
// start with your move
let you = document.getElementById("you");
let ym = null;
/// player choices of weapon
/// not a drop down menu - just click to pick - mobile friendly - no keyboard required
/// rock
let rock = document.getElementById("rock");
rock.addEventListener("click", rockPick);
function rockPick() {
you.innerText = "ROCK";
you.style.borderColor = "red";
ym = "rock";
}
/// paper
let paper = document.getElementById("paper");
paper.addEventListener("click", paperPick);
function paperPick() {
you.innerText = "PAPER";
you.style.borderColor = "white";
ym = "paper";
}
/// scissors
let scissors = document.getElementById("scissors");
scissors.addEventListener("click", sciPick);
function sciPick() {
you.innerText = "SCISSORS";
you.style.borderColor = "gray";
ym = "scissors";
}
/// lizard
let lizard = document.getElementById("lizard");
lizard.addEventListener("click", lizardPick);
function lizardPick() {
you.innerText = "LIZARD";
you.style.borderColor = "green";
ym = "lizard";
}
/// spock
let spock = document.getElementById("spock");
spock.addEventListener("click", spockPick);
function spockPick() {
you.innerText = "SPOCK";
you.style.borderColor = "blue";
ym = "spock";
}
/// click a button after you pick your weapon for computers move
// pick a random number to get a random computer move
function randomMove() {
switch (Math.floor(Math.random() * 5)) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
case 3:
return "spock";
case 4:
return "lizard";
}
}
let shoot = document.getElementById("shoot");
shoot.addEventListener("click", rpsls);
let puter = document.getElementById("comp");
function rpsls(e) {
// keep window open don't re-load page
e.preventDefault();
if (you.innerText === "choice") {
alert("please choose your move first");
} else {
let cm = randomMove();
puter.innerText = cm;
if (cm === "rock") {
puter.style.borderColor = "red";
} else if (cm === "paper") {
puter.style.borderColor = "white";
} else if (cm === "scissors") {
puter.style.borderColor = "gray";
} else if (cm === "lizard") {
puter.style.borderColor = "green";
} else if (cm === "spock") {
puter.style.borderColor = "blue";
} else {
puter.style.borderColor = "black";
}
compare(ym, cm);
return cm;
}
}
/// tell you if round is a 'win', 'lose', or 'draw'
let status = document.getElementById("status");
function compare(ym, cm) {
// console.log("compare");
// console.log(ym);
///// compare
/// declare win lose tie - start with tie - base case
if (ym === cm) {
status.innerText = "You Tied - well at least it's not a loss";
scoreT();
// console.log("tie");
// 10 ways to lose - 2 per option - lowercase you - :-(
} else if (ym === "paper" && cm === "scissors") {
status.innerText = "you lost comp scissors cuts your paper.";
scoreL();
} else if (ym === "rock" && cm === "paper") {
status.innerText = "you lost comp paper covers your rock.";
scoreL();
} else if (ym === "lizard" && cm === "rock") {
status.innerText = "you lost comp rock smash your lizard.";
scoreL();
} else if (ym === "spock" && cm === "lizard") {
status.innerText = "you lost comp lizard poisioned your Spock.";
scoreL();
} else if (ym === "scissors" && cm === "spock") {
status.innerText = "you lost comp Spock smashed your scissors.";
scoreL();
} else if (ym === "lizard" && cm === "scissors") {
status.innerText = "you lost comp scissors killed your lizard.";
scoreL();
} else if (ym === "paper" && cm === "lizard") {
status.innerText = "you lost comp lizard ate your paper.";
scoreL();
} else if (ym === "spock" && cm === "paper") {
status.innerText = "you lost comp paper disproved your Spock.";
scoreL();
} else if (ym === "rock" && cm === "spock") {
status.innerText = "you lost comp Spock vaporized your rock.";
scoreL();
} else if (ym === "scissors" && cm === "rock") {
status.innerText = "you lost comp rock broke your scissors.";
scoreL();
}
// 10 ways to win now - upper case You :-)
else if (ym === "scissors" && cm === "paper") {
status.innerText = "You Won, Scissors cuts comp paper!";
scoreW();
} else if (ym === "scissors" && cm === "lizard") {
status.innerText = "You Won, Scissors decaps comp lizard!";
scoreW();
} else if (ym === "rock" && cm === "scissors") {
status.innerText = "You Won, Rock smashes comp scissors!";
scoreW();
} else if (ym === "rock" && cm === "lizard") {
status.innerText = "You Won, Rock flattens comp lizard!";
scoreW();
} else if (ym === "paper" && cm === "spcok") {
status.innerText =
"You Won, your Paper disproved comp Spock - nice research!";
scoreW();
} else if (ym === "paper" && cm === "rock") {
status.innerText =
"You Won, your Paper covered comp rock, and other good topics!";
scoreW();
} else if (ym === "lizard" && cm === "spock") {
status.innerText = "You Won, your Lizard posioned comp Spock.";
scoreW();
} else if (ym === "lizard" && cm === "paper") {
status.innerText =
"You Won, your Lizard ate the comp paper! .... nom nom nom ...";
scoreW();
} else if (ym === "spock" && cm === "rock") {
status.innerText =
"You Won, your Spock vaporized comp rock! ... pew pew pew ...";
scoreW();
} else if (ym === "spock" && cm === "scissors") {
status.innerText =
"You Won, your Spock smashed comp scissors! - May you live long and prosper!";
scoreW();
}
}
//// might as well keep score if you're playing
let win = document.getElementById("win");
let lose = document.getElementById("lose");
let tie = document.getElementById("tie");
w = 0;
l = 0;
t = 0;
/// if it's a tie
function scoreT() {
t += 1;
tie.innerText = t;
}
/// if its a loss
function scoreL() {
l += 1;
lose.innerText = l;
}
/// if you win - yeah right - you won't.
function scoreW() {
w += 1;
win.innerText = w;
}
import random
import sys
# lets zazz it up here and make a rock paper scissors
# with lizard & spock version
wins = 0
losses = 0
ties = 0
while True: # The main game loop.
print('%s Wins, %s Losses, %s Ties' % (wins, losses, ties))
while True: # The player input loop.
print('Enter your move: (ro)ck (pa)per (sc)issors (li)zard (sp)ock or (q)uit')
playerMove = input()
if playerMove == 'q':
print('thanks for playing your final score was: ')
print('%s Wins, %s Losses, %s Ties' % (wins, losses, ties))
sys.exit() # Quit the program.
if playerMove in['ro','pa','sc','li','sp','r','p','s','l'] :
break # Break out of the player input loop.
print('Type one of ro, pa, sc, li, so, or q.')
# Display what the player chose:
if playerMove == 'ro':
print('ROCK versus...')
elif playerMove == 'pa':
print('PAPER versus...')
elif playerMove == 'sc':
print('SCISSORS versus...')
elif playerMove == 'li':
print('LIZARD versus...')
elif playerMove == 'sp':
print('SPOCK versus...')
# Display what the computer chose:
randomNumber = random.randint(1, 5)
if randomNumber == 1:
computerMove = 'ro'
print('ROCK')
elif randomNumber == 2:
computerMove = 'pa'
print('PAPER')
elif randomNumber == 3:
computerMove = 'sc'
print('SCISSORS')
elif randomNumber == 4:
computerMove = 'li'
print('LIZARD')
elif randomNumber == 5:
computerMove = 'sp'
print('SPOCK')
# Display and record the win/loss/tie:
# start with a tie
if playerMove == computerMove:
print('It is a tie!')
ties = ties + 1
# ways you win
# 2 ways to win with each option
# - 10 total outcomes
# - 6 outcomes if classic Rock Paper Scissors
elif playerMove == 'ro' and computerMove == 'sc':
print('You win! rock crushes scissors')
wins = wins + 1
elif playerMove == 'ro' and computerMove == 'li':
print('You win! rock smashes lizard')
wins = wins + 1
elif playerMove == 'pa' and computerMove == 'ro':
print('You win! paper covers rock')
wins = wins + 1
elif playerMove == 'pa' and computerMove == 'sp':
print('You win! paper disproves Spock')
wins = wins + 1
elif playerMove == 'sc' and computerMove == 'pa':
print('You win! scissors cuts paper')
wins = wins + 1
elif playerMove == 'sc' and computerMove == 'li':
print('You win! scissors decapitates lizard')
wins = wins + 1
elif playerMove == 'li' and computerMove == 'sp':
print('You win! lizard poisions Spock')
wins = wins + 1
elif playerMove == 'li' and computerMove == 'pa':
print('You win! lizard eats paper')
wins = wins + 1
elif playerMove == 'sp' and computerMove == 'sc':
print('You win! Spock smashes scissors')
wins = wins + 1
elif playerMove == 'sp' and computerMove == 'ro':
print('You win! Spock vaporizes rock')
wins = wins + 1
# now the 10 losing scenarios 2 for each weapon choice
# I could just put elif print you lose losses +1 .... but
# it's good to know how you lost to better prepare and win next time
elif playerMove == 'ro' and computerMove == 'pa':
print('You lose! computer paper covered your rock')
losses = losses + 1
elif playerMove == 'ro' and computerMove == 'sp':
print('You lose! computer Spock vaporized your rock')
losses = losses + 1
elif playerMove == 'pa' and computerMove == 'sc':
print('You lose! computer scissors cut your paper')
losses = losses + 1
elif playerMove == 'pa' and computerMove == 'li':
print('You lose! computer lizard ate your paper')
losses = losses + 1
elif playerMove == 'sc' and computerMove == 'ro':
print('You lose! computer rock smashed your scissors')
losses = losses + 1
elif playerMove == 'sc' and computerMove == 'sp':
print('You lose! computer Spock smashed your scissors')
losses = losses + 1
elif playerMove == 'li' and computerMove == 'ro':
print('You lose! computer rock smashed your lizard')
losses = losses + 1
elif playerMove == 'li' and computerMove == 'sc':
print('You lose! computer scissors decapitated your lizard')
losses = losses + 1
elif playerMove == 'sp' and computerMove == 'pa':
print('You lose! computer paper disproved your Spock')
losses = losses + 1
elif playerMove == 'sp' and computerMove == 'li':
print('You lose! computer lizard posioned your Spock')
losses = losses + 1
import java.util.*;
/**
* RPS Refactoring
**/
public class RPSfinal {
public static final String TITLE = "RPSLX";
public static final String GUESSES = "rpslx";
public static String[] winners = {"rs", "sp", "pr", "px", "rl", "xs", "lx", "sl", "lp", "xr"};
public static final String STOP = "stop";
private static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
int win = 0;
int lose = 0;
int draw = 0;
Arrays.sort(winners);
for (String userHand = getUserThrow();
!userHand.equals(STOP);
userHand = getUserThrow()) {
String myHand = getComputerThrow();
String combined = userHand + myHand;
if (compareThrows(userHand, myHand)) {
win++;
showScore(win, lose, draw, "\nYou Won!", "Score: wins:");
} else if (myHand.equals(userHand)) {
draw++;
showScore(win, lose, draw, "\nIt's a tie!", "Score: wins:");
} else {
lose++;
showScore(win, lose, draw, "\nI win!", "Score: wins:");
}
}
showScore(win, lose, draw, "\nThanks for playing!", "FINAL SCORE: wins:");
}
private static boolean compareThrows(String userHand, String myHand) {
String combined = userHand + myHand;
int result = Arrays.binarySearch(winners, combined);
return result >= 0;
}
private static void print(Object o) {
System.out.println(o);
}
private static void showScore(int win, int lose, int draw, String s, String s2) {
print(s);
print(s2 + win + " lose: " + lose + " draw: " + draw);
}
private static String getComputerThrow() {
int rnd = myRandom(0, GUESSES.length() - 1);
String myHand = GUESSES.substring(rnd, rnd + 1);
print("I throw > " + myHand);
return myHand;
}
private static int myRandom(int min, int max) {
return (int) Math.floor(Math.random() * (max - min)) + min;
}
private static String getUserThrow() {
print("\nLet's play " + TITLE);
print("Throw one of " + GUESSES + "> ");
String userHand = getUserThrowNormalized();
while (!userHand.equals(STOP) && !GUESSES.contains(userHand)) {
print("\nPlease enter a valid throw");
print("\nLet's play RPS"); // this looks familiar
print("Throw one of 'rps' > ");// this looks familiar
userHand = getUserThrowNormalized();
}
return userHand;
}
private static String getUserThrowNormalized() {
return scanner.nextLine().trim().toLowerCase();
}
}
// This code is contributed by Greg Smith
To get started using these click the links at the top of the page to download Python or Java. There is no requirement to download or install JavaScript to use it. You will want a code editor program to interact with these languages like VSCode, Atom, Sublime, Intelli-J, or Eclipse which all have free versions or trials. There are many courses and tutorials out there from the creators of these programs as well as by those who use them.
Well now you know that these languages might appear very different but at their core share more similarities than differences. Modifying and manipulating words and numbers is essentially all any computer program does. While they might have slightly different approaches, and some programmers swear by one and curse the other. Honestly there is not one clear hands-down winner or loser. You can use Node / Express (JavaScript) to make back-ends for websites or Django (Python) or Java DB / Springboot (Java). While they might have different ways of completing the tasks assigned to them they are all, like me, quite capable of getting the job done.